All SkinHelpDesk APIs are available on RapidAPI
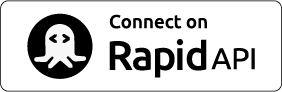
All SkinHelpDesk APIs accept JPEG images submitted as a Base64 encoded string without the header. You can download this simple HTML file to test the APIs with a webcam after adding the API key (See comments in the file). The implementation depends on the programming language used by the client application. Here are some examples of how to do this in javascript, java and python:
Javascript
function getBase64Image(img) {
// Create an empty canvas element
var canvas = document.createElement("canvas");
canvas.width = img.width;
canvas.height = img.height;
// Copy the image contents to the canvas
var ctx = canvas.getContext("2d");
ctx.drawImage(img, 0, 0);
// Get the data-URL formatted image
var dataURL = canvas.toDataURL("image/jpeg");
return dataURL.replace(/^data:image\/(png|jpg|jpeg);base64,/, "");
}
You can read more about customization options here
Java
byte[] fileContent = FileUtils.readFileToByteArray(new File(filePath));
String encodedString = Base64.getEncoder().encodeToString(fileContent);
Python
import base64
with open("yourfile.ext", "rb") as image_file:
encoded_string = base64.b64encode(image_file.read())
Image requirements
Image acquisition requirements vary according to the API used. Please refer to the API description for more details.